One Tap Instead of Dropdown
Dropdowns require multi-taps or -clicks. Providing succinct text or an easily-recognizable image or icon to tap is a friendly gesture.
The ease might not be actively appreciated, because the user generally doesn't know a dropdown would otherwise have been required. But, and this is important, they also don't feel the form-abandonment frustration they might have experienced had they actually encountered a dropdown.
It works like this:
-
The user taps on the item of their choice and a value representing the choice is inserted into a type="hidden" form field.
-
When the form is submitted, the hidden field value is submitted with all the other form information.
Below is an illustration with tappable items. Try it by tapping or clicking on one. Than another.
For this illustration, the type="hidden" field has been changed to type="text" to show the value change when a different item is tapped (located immediately below the tappable items).

Willmaster
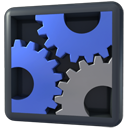
Software
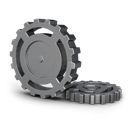
Custom
Implementing the One Tap Functionality
The Hidden Field
The hidden field is a normal type="hidden" form field. It needs to have an id value. Example:
<input type="hidden" id="hidden_field_id">
Change the hidden_field_id
id value to your preference. Whatever id value you assign to the hidden field, that id value will be specified in the JavaScript provided further below.
The hidden field needs to be within the form so it's included with the form data when the form is submitted. (The rest of the implementation can be anywhere on the page.)
The Tappable Items
The tappable items contain text/images to tap on.
First, I'll provide minimum code, only what's absolutely required for functionality. The reason is to make the more elaborate code used in the earlier illustration easier to assimilate.
This code provides three tappable items, each with minimum code.
<div id="one" onclick="SelectThisOne(this)"> Text/image for first tappable item. </div> <div id="two" onclick="SelectThisOne(this)"> Text/image for second tappable item. </div> <div id="three" onclick="SelectThisOne(this)"> Text/image for third tappable item. </div>
The minimum HTML code:
Each of the three items must have these:
-
A
div
with anid
value. In the above code, theid
values areone
,two
, andthree
. The JavaScript (further below) will be customized so it knows what id values to expect.(It doesn't have to be an
div
container; it can be any other container likespan
,td
, orpre
.) -
An
onclick
attribute with the exact value in the example:onclick="SelectThisOne(this)"
Tapping on the
div
launches the custom JavaScriptSelectThisOne()
function, which provides identification of thediv
. -
Text and/or image for the user to tap.
The above represents the minimum HTML code.
The number of tappable items may be more or less than three.
The more elaborate HTML code:
Now, the code used in the earlier illustration.
First, the CSS style sheet. Specifying a class name instead of lots of inline CSS makes the tappable item div
areas seem less cluttered.
<style type="text/css"> .exampleselection { float:left; border:1px solid blue; padding:13px 0 13px 0; border-radius:3pt; text-align:center; width:100px; height:100px; box-sizing:border-box; margin:0 3px 3px 0; } </style>
Having seen the minimum HTML code, you understand that all the above CSS code is optional. Among other things, the CSS sizes the tappable items and puts a border around them. It also floats the items left for width responsiveness.
Add, remove, and change the CSS styles according to your preferences.
Now, the code for the tappable items.
<div id="one" onclick="SelectThisOne(this)" class="exampleselection"> <img src="https://www.willmaster.com/images/wmlogo_icon.gif" style="max-height:50px; max-width:50px;" alt="Willmaster logo"> <br>Willmaster </div> <div id="two" onclick="SelectThisOne(this)" class="exampleselection"> <img src="https://www.willmaster.com/images/icons/software-icon.png" style="max-height:50px; max-width:50px;" alt="Willmaster logo"> <br>Software </div> <div id="three" onclick="SelectThisOne(this)" class="exampleselection"> <img src="https://www.willmaster.com/images/icons/custom-software-icon.png" style="max-height:50px; max-width:50px;" alt="Willmaster logo"> <br>Custom </div> <div style="clear:left;"></div>
As you scan the code, you'll notice it's similar to the minimum HTML code provided earlier.
This code for each tappable item has a class name to implement the CSS style specified in the style sheet. It also contains an image in addition to text.
Below the set of tappable items is an empty div to clear the float:left
style incorporated from the style sheet.
The id
values one
, two
, and three
will be provided to the JavaScript (further below) so it knows what id values to expect.
All that's left now is the JavaScript.
The JavaScript
The JavaScript has two custom functions, SelectThisOne()
and PowerhouseStyleChange()
.
That second one, PowerhouseStyleChange()
, is copied straight out of the Powerhouse CSS Style Changer article. It needs no customization.
The other function, SelectThisOne()
, however does need customization — in five places.
Here is the JavaScript with both functions. Customization notes follow.
<script type="text/javascript"> function SelectThisOne(d) { var valueID = "hidden_field_id"; var idList = Array("one", "two", "three"); var valueList = Array("Willmaster.com Website", "Software", "Custom Software"); var selectedCSS = "border:3px solid blue; opacity:1.0"; var unselectedCSS = "border:1px solid blue; opacity:0.5"; var thisID = d.id; for( var i=0; i<idList.length; i++ ) { var style = unselectedCSS; if( thisID == idList[i] ) { style = selectedCSS; document.getElementById(valueID).value = valueList[i]; } PowerhouseStyleChange(idList[i],style); } } function PowerhouseStyleChange(idvalue,style) { // Version 1.0, March 14, 2017. // Version 1.1, November 10, 2017 (removed .replace(...) from last code line) // Will Bontrager Software LLC - https://www.willmaster.com/ var id = document.getElementById(idvalue); var ta = style.split(/\s*;\s*/); for( var i=0; i<ta.length; i++ ) { var tta = ta[i].split(':',2); var ttaa = tta[0].split('-'); if( ttaa.length > 1 ) { for( var ii=1; ii<ttaa.length; ii++ ) { ttaa[ii] = ttaa[ii].charAt(0).toUpperCase() + ttaa[ii].slice(1); } } tta[0] = ttaa.join(''); id.style[tta[0]] = tta[1]; } } </script>
Customization notes:
Five lines of the SelectThisOne()
JavaScript function need to be customized. The values currently in the function are the values used by the earlier illustration.
-
Find this line in the function.
var valueID = "hidden_field_id";
hidden_field_id
is the id value of The Hidden Field for containing the value of whichever tappable item is tapped.In the JavaScript function, replace
hidden_field_id
with the id value of your type="hidden" form field. -
Find this line in the function.
var idList = Array("one", "two", "three");
one
,two
, andthree
are the id values of the three tappable items in the earlier illustration. Your implementation may have as many or as few tappable items as required.In the JavaScript function, replace
"one", "two", "three"
with the id values of your tappable items. Each id value is bounded with quotation marks. The id values are separated with a comma and optional space(s). -
Find this line in the function.
var valueList = Array("Willmaster.com Website", "Software", "Custom Software");
When a tappable item is tapped on, the tappable item's value is stored in the hidden form field. See customization notes #1.
Willmaster.com Website
,Software
, andCustom Software
are the customization item values in the earlier illustration. The customization item values correspond to the customization item id values listed in customization notes #2.The value corresponding to the tapped item will be stored in the hidden form field.
In the JavaScript function, replace
"Willmaster.com Website", "Software", "Custom Software"
with the tappable item values that correspond to the list of id values in the list dealt with at customization notes #2.. Each tappable item value is bounded with quotation marks. The tappable item values are separated with a comma and optional space(s). -
Find this line in the function.
var selectedCSS = "border:3px solid blue; opacity:1.0";
border:3px solid blue; opacity:1.0
is the CSS style to apply to a tappable item when the item is tapped. Any CSS styles may be specified here.In the JavaScript function, replace
border:3px solid blue; opacity:1.0
with the CSS styles for the tappable item that is tapped. -
Find this line in the function.
var unselectedCSS = "border:1px solid blue; opacity:0.5";
border:1px solid blue; opacity:0.5
is the CSS style to apply to a tappable item when a different tappable item is tapped. Any CSS styles may be specified here.In the JavaScript function, replace
border:1px solid blue; opacity:0.5
with the CSS styles for tappable items that have not been tapped.
When the JavaScript has been customized, paste it into the web page source code. It can be pasted anywhere that JavaScript can run. Near the bottom of the page, above the cancel </body>
tag generally is a good place.
The JavaScript may also be imported from an external file.
One quick tap or click is so very much more convenient than the multiple taps and perhaps scrolling that are required for a dropdown selection.
(This article first appeared in Possibilities newsletter.)
Will Bontrager