PHP 'Headers Already Sent' Error Message
When a browser requests a web page from the server, the server responds with the page. The web page is the content. Before the browser gets the content itself, it gets information about the content — the kind of content, whether it's compressed, any cookies that apply to the page, cache control, and other items of information that the browser may need to present the web page to you.
The stuff sent before the content is the response header data. HTTP Headers has an extensive list of possible response header data that might be sent.
The response header data that is sent before the content is different than the web page head
area. The web page head
area is part of the web page content. Response header data is sent before the content.
Generally, you don't have to worry about all that. The server automatically sends some of the header data. The PHP processor (the server software that processes your PHP code) sends some, too. In only special cases do you need to send header data to the browser yourself.
The header data will always be sent before the content.
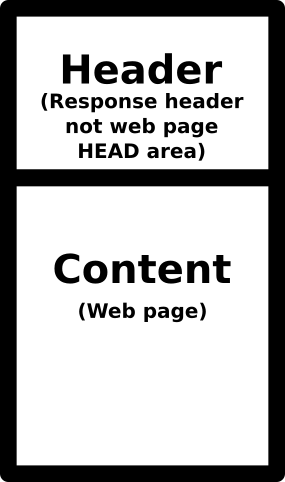
Once any part of the content has been sent to the browser, no more header data will be accepted.
You get the PHP "headers already sent" error message when you try to send header data after any content has been sent. There may be an alternate way to send the data to the browser when that error message is encountered.
The PHP header() function can be used to send header data to the browser. Code to cause a browser redirect is an example.
It is possible to set cookies with the header() function, because they are actually header data. Generally, however, the setcookie() function is preferred for setting cookies. Function setcookie() sets cookies as header lines, but first it automatically conforms the expiration date and does other formatting.
When you get a PHP 'headers already sent' error message, PHP code tried to send header data after headers had already been sent. Either the code must be fixed or an alternative must be implemented — assuming the header data is indeed important.
To fix the code, ensure the header() or setcookie() functions are used before any non-header content is sent to the browser. Sometimes it's an easy or obvious fix. Sometimes the fix is elusive. But it must be accomplished if the code is to be fixed.
For some header data, there are alternatives.
-
The header line to redirect the browser to another URL can also be done with HTML and with JavaScript.
-
The header line to set a cookie can also be done with JavaScript.
Further below is example code for each of the mentioned alternatives.
PHP code checks to see if headers have already been sent. If already sent, PHP publishes the alternate code to the web page.
(1)
If the browser needs to be redirected:
Let's assume you want to redirect the browser. Using the PHP header() function is fastest because the redirect takes place before any web page content is sent to the browser. If header data has already been sent, there are two alternatives. One is to redirect with HTML and the other is to redirect with JavaScript.
This code will redirect the browser with HTML if header data has already been sent.
$URL = "http://example.com/page.php"; // The URL to redirect to. if( headers_sent() ) { echo '<meta http-equiv="refresh" content="0; url=$URL">'; } else { header("Location: $URL"); }
The variable $URL
contains the URL for the browser to be redirected to.
How it works:
If headers have already been sent, PHP publishes the meta http-equiv="refresh"
meta tag (blue in above code) to tell the browser to refresh the web page with the indicated URL. Otherwise, PHP publishes the data as a header (red in above code).
This code will redirect the browser with JavaScript if header data has already been sent.
$URL = "http://example.com/page.php"; // The URL to redirect to. if( headers_sent() ) { echo("<script>location.href='$URL'</script>"); } else { header("Location: $URL"); }
As before, the variable $URL
contains the URL for the browser to be redirected to.
How it works:
If headers have already been sent, PHP publishes the JavaScript to redirect the browser (blue in above code). Otherwise, PHP publishes the data as a header (red in above code).
(2)
If a cookie needs to be set:
Let's assume you want to set a cookie. Below is a way to do it depending on whether headers have already been sent. You'll notice it has more code than the previous illustration. Notes follow the code.
$cookieName = 'aCookie'; // Required to have a value. // Optionally, values for the next three variables may be a null string, like "" $cookieValue = 'Once Upon a Time'; $cookiePath = '/'; $cookieDomain = preg_replace('!\:.*$!','',$_SERVER['HTTP_HOST']); // Optionally, values for the next three variables may be 0 or false $cookieExpiration = time() + (3*24*60*60); // (days*hours*minutes*seconds). $cookieHTTPonly = false; $cookieSecure = true; if($cookieDomain and substr_count($cookieDomain,'.')<2){$cookieDomain=".$cookieDomain";} if( headers_sent() ) { $cookieChunks = array(); if($cookieExpiration>0) { $cookieChunks[]='expires='.gmdate('Y-m-d H:i:s',$cookieExpiration).' GMT'; } if($cookiePath) { $cookieChunks[]='path='.$cookiePath; } if($cookieDomain) { $cookieChunks[]='domain='.$cookieDomain; } if($cookieSecure) { $cookieChunks[]='secure'; } if(count($cookieChunks)) { $cookieInformation = implode('; ',$cookieChunks); echo "<script>document.cookie='$cookieName='+encodeURIComponent('$cookieValue')+'; $cookieInformation';</script>"; } else { echo "<script>document.cookie='$cookieName='+encodeURIComponent('$cookieValue');</script>"; } } else { setcookie($cookieName,$cookieValue,$cookieExpiration,$cookiePath,$cookieDomain,$cookieSecure,$cookieHTTPonly); }
How the above source code works:
The first part of the code contains 7 variables. Only the first one ($cookieName
) must contain a value of one or more characters. The other 6 may contain a null string or a 0
/false
value. The comments in the code tell you which values may optionally be a null string (quotation marks with nothing between them, like ""
or ''
) and which values may optionally be 0
or false
.
If headers have already been sent, PHP publishes the lines of code to set the cookie with JavaScript (blue in above code). Otherwise, the cookie is set with the PHP setcookie() function (red in above code).
The alternatives in this article can reduce coding time. Instead of extensive revamping of code, it may be appropriate to use the alternative.
Other alternatives may be useful, too. Check if headers have been sent and, if yes, publish the alternative.
This article first appeared with an issue of the Possibilities newsletter.
Will Bontrager