Compress a File
Ever wanted to ZIP a file on your server?
Here's a tool to do exactly that.
Grab it. Put the PHP file on your server. And you're good to go.
This is something I've been wanting for quite a while.
Generally, when projects are completed, I zip up the files for delivery to the clients.
Well, when I want to zip up a single file in a directory that contains other files, my good old workhorse Directory Zip won't work. It zips entire directories, not individual files. (Directory Zip is a WebSite's Secret exclusive.)
So what I've been having to do is create a directory, put the file into it all by itself, then zip the directory.
It takes only few minutes to do it. Still, every time I wish I had a single-file zipper.
A Database Back Up and Restore software purchase last week made it imperative to go ahead and program the zipper.
The customer has huge MySQL database tables – pretty close to a gigabyte of files with a full backup.
The backup files are plain text CSV files. Zipping them up and then deleting the original will restore a lot of server space to the hosting account.
Here is the result of the first phase of the project – a PHP script to compress a file and, optionally, delete the original.
Copy the source code in this box, save it as compressfile.php, and upload it to your server. (It may have a different file name, if you wish, so long as it ends with ".php")
<?php /* Compress a File Version 1.0 November 4, 2013 Will Bontrager Software LLC https://www.willmaster.com/ Copyright 2013 Will Bontrager Software LLC This software is provided "AS IS," without any warranty of any kind, without even any implied warranty such as merchantability or fitness for a particular purpose. Will Bontrager Software LLC grants you a royalty free license to use this software provided this notice appears on all copies. */ $HasOne = true; $Choices = array(); $Zlib = $Zip = false; $doZlib = $doZip = false; $from = $to = $unlink = ''; $Process = false; $Error = array(); if( function_exists('gzopen') ) { $Zlib = true; } if( class_exists('ZipArchive') ) { $Zip = true; } if( $Zip ) { $Choices[] = '<input type="radio" name="which" value="Zip" checked="checked"> Zip (*.zip) compression'; } if( $Zlib ) { $Choices[] = '<input type="radio" name="which" value="Zlib"' . ($Zip?'':' checked="checked"') . '> Zlib (*.gz) compression'; } if( ! ($Zlib or $Zip) ) { $HasOne = false; $Choices[] = 'Unfortunately, neither Zlib (*.gz) nor Zip (*.zip) compression is available.'; } if( isset($_POST['submitter']) ) { $Process = true; $from = stripslashes(trim($_POST['from'])); $to = stripslashes(trim($_POST['to'])); if( ! ($from and $to) ) { $Error[] = 'Both "Location of the file to compress" and "Location for the compressed file" must have valid data to do a compression.'; $Process = false; } else { $from = preg_replace('(^\Q' . $_SERVER['DOCUMENT_ROOT'] . '\E)','',$from); $to = preg_replace('(^\Q' . $_SERVER['DOCUMENT_ROOT'] . '\E)','',$to); $from = $_SERVER['DOCUMENT_ROOT'] . $from; $to = $_SERVER['DOCUMENT_ROOT'] . $to; } if( isset($_POST['which']) and $_POST['which']=='Zlib' ) { $doZlib = true; } if( isset($_POST['which']) and $_POST['which']=='Zip' ) { $doZip = true; } if( $doZlib ) { if( ! preg_match('/\.gz$/',$to) ) { $to .= '.gz'; } } elseif( $doZip ) { if( ! preg_match('/\.gz$/',$to) ) { $to .= '.zip'; } } else { $Error[] = 'Something is awry with the compression method selection. Verify a compression method is checked.'; $Process = false; } if( isset($_POST['unlink']) and $_POST['unlink'] == 'yes' ) { $unlink = true; } } if( $Process ) { if( $doZlib ) { CompressZlib($from,$to,$unlink); } elseif( $doZip ) { CompressZip($from,$to,$unlink); } } function CompressZlib($from,$to,$unlink) { global $Error; if( ! ($gz = gzopen($to,'w9')) ) { return NoCanCompress($to); } gzwrite($gz,file_get_contents($from)); gzclose($gz); if( $unlink ) { unlink($from); } } # function CompressZlib() function CompressZip($from,$to,$unlink) { global $Error; $zip = new ZipArchive(); if( $zip->open($to, ZipArchive::CREATE) !== TRUE ) { return NoCanCompress($to); } $zip->addFile( $from, preg_replace('!^.*/!','',$from) ); $zip->close(); if( $zip->status > 0 ) { return NoCanCompress($to); } if( $unlink ) { unlink($from); } } # function CompressZip() function NoCanCompress($to) { global $Error; $ts = preg_replace('!/[^/]+$!','',$to); $ts = preg_replace('(^\Q' . $_SERVER['DOCUMENT_ROOT'] . '\E)','',$ts); $Error[] = "Either the $ts directory doesn't exist or it has insufficient permissions."; $Error[] = 'For permissions, try giving the directory 777 permissions.'; return true; } # function NoCanCompress() ?><!DOCTYPE html> <html lang="en"> <head> <meta http-equiv="Content-Type" content="text/html;charset=utf-8" > <title>Compress a File</title> <style type="text/css"> body { margin:0; font-family:sans-serif; font-size:14px; text-align:left; } th { font-size:.8em; } p, li, td { font-size:1em; line-height:120%; } h1 { font-size:1.8em; } h2 { font-size:1.6em; } h3 { font-size:1.4em; } h4 { font-size:1.2em; } h5 { font-size:1em; } a { text-decoration:none; color:#1c5292; font-weight:bold; } a, a img { border:none; outline:none; } #content { margin:0 0 0 150px; padding:75px 0 100px 50px; width:400px; border-left:6px groove #2F83E5; } </style> </head> <body><div id="content"> <div style="position:fixed; left:50px; top:50px;"> <a href="//www.willmaster.com/"> <img src="//www.willmaster.com/images/wmlogo_icon.gif" style="width:50px; height:50px; border:none;" alt="Willmaster logo"> </a> </div> <h1> Compress a File </h1> <form method="post" enctype="multipart/form-data" action="<?php echo($_SERVER['PHP_SELF']); ?>"> <?php if( count($Error) ): ?> <div style="border:3px double red; padding:25px; font-size:14px; color:red;"> <?php echo( implode('<br><br>',$Error) ); ?> </div> <?php endif; ?> <form method="post" enctype="multipart/form-data" action="<?php echo($_SERVER['PHP_SELF']); ?>"> <?php echo '<p>' . implode('<br>',$Choices). '</p>'; ?> <?php if($HasOne): ?> <p> Location of the file to compress.<br> <input type="text" name="from" style="width:392px; border:1px solid black; padding:3px;"> </p> <p> Location for the compressed file (directory must be writable).<br> <input type="text" name="to" style="width:392px; border:1px solid black; padding:3px;"> </p> <p> <input type="checkbox" style="margin:0;" name="unlink" value="yes"> After compressing the file, delete the original. </p> <p> <input type="submit" name="submitter" value="Compress the File" style="width:400px;"> </p> <?php endif; ?> </form> <p style="margin-top:35px; margin-left:-35px;"> Copyright 2013 <a href="//www.willmaster.com/">Will Bontrager Software LLC</a> </p> </div> </body> </html>
After the compressfile.php file is uploaded to your server, type its URL into your browser. You'll see the control panel. Here's a screenshot.
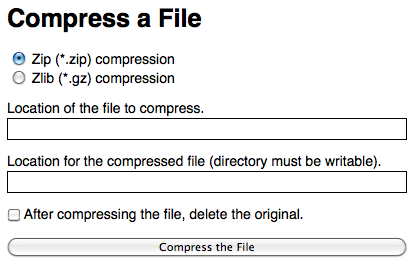
On the control panel, you'll have two, one, or no radio buttons, depending on what's available with the PHP installation your hosting company provides:
-
Two radio buttons — You have a choice of compression method. One will make a .zip compressed file and the other will make a .gz compressed file.
-
One radio button — Only one compression method is available, either .zip file compression or .gz file compression.
Note: Both .zip and .gz decompress with virtually all desktop software designed to decompress files. It doesn't really matter which of these two compression methods you have available.
-
No radio buttons — Unfortunately, your server's PHP installation has neither compression method available.
The two text fields are to specify the file to compress and where to put the compressed file. The locations are specified as URI's – the URL minus the http:// and domain name. From URL http://example.com/file.jpg you get URI /file.jpg
Important note: The directory where the compressed file is to be stored must have sufficient permissions. If 755 doesn't work, try 666 and 766. If neither of those work, give the directory 777 permissions.
On the control panel, you'll see a checkbox to delete the original file after it has been compressed.
Specialized Version
A specialized version of Compress a File for use with Database Back Up and Restore will have these features:
-
Designed to be run with a cron schedule (no control panel).
-
Automatically compress each CSV file in the backup files directory and then delete the original CSV file.
The specialized version will come with Database Back Up and Restore purchases beginning sometime this week. (If you already own Database Back Up and Restore, send me an email and I'll reply with the specialized version attached.)
Will Bontrager