Integrated Uploader
This software was written for a client who needed a quick way to upload images to their website.
The client is completely new to operating a website. Thus, the software was written to be as straight-forward as I could make it. To use the software, they had no need to learn FTP or how to use a website dashboard.
I installed it for the client. It worked as designed.
The software might be good for you or your business, too. If you have technically challenged clients, for example. Or, if you want to have a file upload feature that does not require providing FTP access. Even the technically adept are likely to appreciate it's simplicity.
It is an integrated uploader. It includes the form, the uploading code, and messages about the upload, all in one PHP file.
Here is a screenshot.
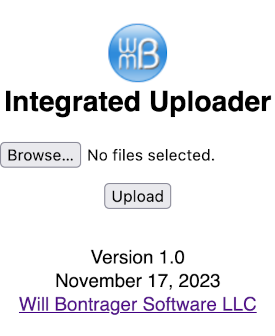
- Tap "Browse"
- Select one or more files
- Tap "Upload"
Here is another screenshot — after 2 files have been selected for uploading.
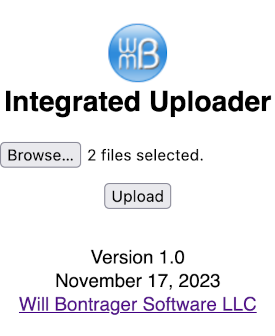
The software is available with this article.
<?php /* Integrated Uploader Version 1.0 November 17, 2023 Will Bontrager Software LLC https://www.willmaster.com/ */ mb_regex_encoding('UTF-8'); mb_internal_encoding('UTF-8'); ini_set('display_errors',1); error_reporting(E_ALL); $IUglobal = array(); $IUglobal['Messages'] = array(); if( isset($_FILES['file_upload']['name'][0]) ) { IUuploadFiles(); } function IUuploadFiles() { global $IUglobal; $countfiles = count($_FILES['file_upload']['name']); for( $upfile=0; $upfile<$countfiles; $upfile++ ) { $f = $fname = $_FILES['file_upload']['name'][$upfile] ? $_FILES['file_upload']['name'][$upfile] : '(blank)'; if( $_FILES['file_upload']['error'][$upfile] ) { $error = IUgetErrorMessage($_FILES['file_upload']['error'][$upfile]); IUstoreMessage("File "<b>$fname</b>" experienced an error: $error"); continue; } $size = $_FILES['file_upload']['size'][$upfile]; $newfname = IU_StoreUploadedFileOnServer($fname,$_FILES['file_upload']['tmp_name'][$upfile]); IUstoreMessage("File "<b>$f</b>" (size <b>$size</b> bytes) was uploaded."); } } #function IUuploadFiles() function IU_StoreUploadedFileOnServer($fname,$fromlocation) { $todirectory = __DIR__; $filelist = array(); foreach( glob("$todirectory/*") as $f ) { $filelist[preg_replace('!^.*/!','',$f)] = true; } if( isset($filelist[$fname]) ) { $ta = explode('.',$fname); $extension = count($ta)>1 ? ('.' . array_pop($ta)) : ''; $fname = implode('.',$ta); $startnumber = 0; $newname = "$fname$extension"; if( isset($filelist[$newname]) ) { while( isset($filelist[$newname]) ) { $startnumber++; $newname = $fname . '-' . $startnumber . $extension; } $fname = $newname; } else { $fname = $newname; } } move_uploaded_file($fromlocation,__DIR__."/$fname"); return($fname); } # function IU_StoreUploadedFileOnServer() function IU_AppendRandomCharacter($s,$howmany=1) { $chars = 'abcdefghijklmnopqrstuvwxyz0123456789'; $topselection = strlen($chars)-1; $cstring = ''; for($i=0; $i<$howmany; $i++) { $cstring .= substr($chars,mt_rand(0,$topselection),1); } return $s . $cstring; } # function IU_AppendRandomCharacter() function IUgetErrorMessage($enum) { switch($enum) { case UPLOAD_ERR_OK /* Value: 0 */ : return 'There is no error, the file uploaded with success.'; case UPLOAD_ERR_INI_SIZE /* Value: 1 */ : return 'The uploaded file exceeds the upload_max_filesize directive in php.ini.'; case UPLOAD_ERR_FORM_SIZE /* Value: 2 */ : return 'The uploaded file exceeds the MAX_FILE_SIZE directive that was specified in the HTML form.'; case UPLOAD_ERR_PARTIAL /* Value: 3 */ : return 'The uploaded file was only partially uploaded.'; case UPLOAD_ERR_NO_FILE /* Value: 4 */ : return 'No file was uploaded.'; #case UPLOAD_ERR_XXXX /* Value: 5 */ : return 'Undefined.'; case UPLOAD_ERR_NO_TMP_DIR /* Value: 6 */ : return 'Missing a temporary folder. (Hosting company needs to know.)'; case UPLOAD_ERR_CANT_WRITE /* Value: 7 */ : return 'Failed to write file to disk.'; case UPLOAD_ERR_EXTENSION /* Value: 8 */ : return 'A PHP extension stopped the file upload. PHP does not provide a way to ascertain which extension caused the file upload to stop; examining the list of loaded extensions with phpinfo() may help.'; } return 'Undefined error.'; } # function IUgetErrorMessage() function IUstoreMessage($s='') { global $IUglobal; if( empty($s) ) { $s = 'Undefined message.'; } $IUglobal['Messages'][] = $s; return true; } # function IUstoreMessage() ?><!DOCTYPE html> <html lang="en"> <head> <meta http-equiv="Content-Type" content="text/html;charset=UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Integrated Uploader</title> <style type="text/css">html { font-family:sans-serif; font-size:100%; }</style> </head> <body> <form enctype="multipart/form-data" action="<?php echo($_SERVER['PHP_SELF']) ?>" method="post" accept-charset="utf-8"> <div style="display:table; margin:.25in auto; text-align:center;"> <h2><img src="https://www.willmaster.com/images/wmlogo_icon.gif" alt="logo"><br>Integrated Uploader</h2> <?php if(count($IUglobal['Messages'])): ?> <div style="margin-bottom:.25in; text-align:left; border:1px solid blue; padding:1em; border-radius:.7em;"> – <b>Message Box</b> – <ul style="margin-bottom:0;"> <li> <?php echo(implode('</li><li style="margin-top:.5em;">',$IUglobal['Messages'])); ?> </li> </ul> </div> <?php endif; ?> <input type="file" accept=".png,.pdf" name="file_upload[]" multiple><br> <input type="submit" value="Upload" style="margin-top:1em;"> <p style="margin-top:2em; line-height:125%;"> Version 1.0<br>November 17, 2023<br><a href="https://www.willmaster.com/">Will Bontrager Software LLC</a> </p> </div> </form> </body> </html>
No customization is required for installing the software. Just put the one PHP script on your server and it is ready to use.
The script stores uploaded files in the same directory on the server as where the script is running. Therefore, the script needs to be installed in the directory where you want the files to end up at.
Here are the installation steps.
- Save the source code as
uploader.php
or other*.php
file name. - Upload
uploader.php
to your server in the directory where the files shall be stored when the script is used. - Make a note of the URL where
uploader.php
was installed.
Once uploaded, send the URL to the folks who will be using it. (Or, use it yourself; it is just as easy to use for people who are tech knowledgable as it is for those who are not.)
This software is handy for people who:
- Don't have the immediate time available to learn new file transferring software.
- Want to let an acquaintance upload a file but don't want to provide FTP passwords.
Integrated Uploader can be used when attaching a file to an email is inappropriate.
It can be used to upload content-sensitive files (use HTTPS instead of HTTP).
It's a one-file installation, no customization, so it's a quick install for when the need for it suddenly arises.
(This content first appeared in Possibilities newsletter.)
Will Bontrager