Time Elapsed
This article comes with a no-customization-required PHP script to calculate the time elapsed between two different points.
If you need to time your projects, whether for billing or to make better estimates in the future, the script can come in handy.
If you need to keep a record of various time periods, software that will keep a log may be preferred. But for a quick calculation, the script in this article can work. Keep it handy at your website. (You do have a private /mytools or similar directory, right?)
The original script was created for myself to use when calculating the billing time of a quick project. It's quick. It replies with various timeframes, as appropriate (total seconds, minutes and seconds, also hours, and days).
Calculating time differences can be a bit tricky. Three number bases are involved: Base 60 for minutes and seconds, base 24 for hours, and base 10 for the rest of the calculations. The ease of getting the calculations wrong is why this software was built.
The screenshot below contains the calculation results of time that elapsed. The bottom part of the screenshot shows the form to be used for the next calculation.
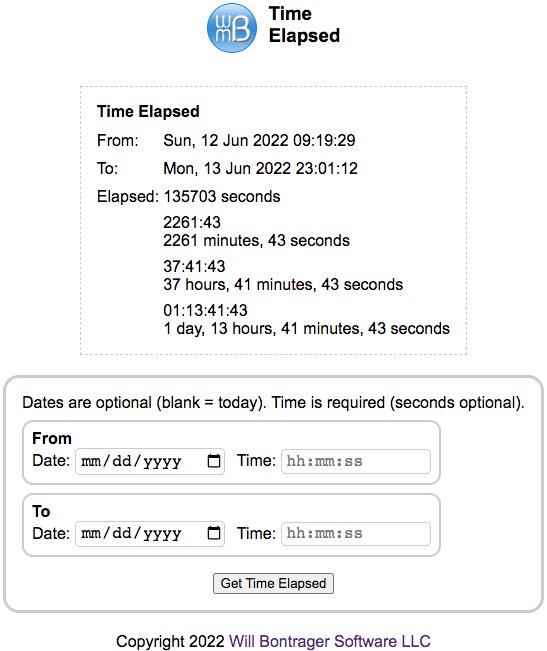
The software is easy and intuitive to use. Nevertheless, after the source code, below, I'll mention a few things so you'll know what you're getting.
<?php /* Time Elapsed Version 1.2 June 14, 2022 Will Bontrager Software LLC https://www.willmaster.com/ */ #if( ! ini_get('date.timezone') ) { date_default_timezone_set('America/Chicago'); } if( count($_POST) ) { $ta = explode(' ',date('Y m d H i s')); $today = array('y'=>intval($ta['0']),'m'=>intval($ta['1']),'d'=>intval($ta['2'])); $now = array('y'=>intval($ta['3']),'m'=>intval($ta['4']),'d'=>intval($ta['5'])); $_POST['fDate'] = ConformInputDate($_POST['fDate']); $_POST['tDate'] = ConformInputDate($_POST['tDate']); $_POST['fTime'] = ConformInputTime($_POST['fTime']); $_POST['tTime'] = ConformInputTime($_POST['tTime']); if( ! $_POST['fDate'] ) { $_POST['fDate'] = $today; } if( ! $_POST['tDate'] ) { $_POST['tDate'] = $today; } if( ! $_POST['fTime'] ) { $_POST['fTime'] = $now; } if( ! $_POST['tTime'] ) { $_POST['tTime'] = $now; } $_POST['fStamp'] = mktime($_POST['fTime']['h'],$_POST['fTime']['m'],$_POST['fTime']['s'],$_POST['fDate']['m'],$_POST['fDate']['d'],$_POST['fDate']['y']); $_POST['tStamp'] = mktime($_POST['tTime']['h'],$_POST['tTime']['m'],$_POST['tTime']['s'],$_POST['tDate']['m'],$_POST['tDate']['d'],$_POST['tDate']['y']); if( $_POST['fStamp'] > $_POST['tStamp'] ) { $i = $_POST['fStamp']; $_POST['fStamp'] = $_POST['tStamp']; $_POST['tStamp'] = $i; } $_POST['fPostTime'] = preg_replace('/ [\+\-]?\d+$/','',date('r',$_POST['fStamp'])); $_POST['tPostTime'] = preg_replace('/ [\+\-]?\d+$/','',date('r',$_POST['tStamp'])); $_POST['seconds'] = $_POST['tStamp'] - $_POST['fStamp']; $_POST['minutes'] = false; $_POST['hours'] = false; $_POST['days'] = false; $minute = 60; if( $_POST['seconds'] >= $minute ) { $m = intval($_POST['seconds']/$minute); $mm = $m < 10 ? "0$m" : $m; $s = $_POST['seconds']-($minute*$m); $ss = $s < 10 ? "0$s" : $s; $_POST['minutes'] = "$mm:$ss<br>{$m} minute".($m==1?'':'s').", {$s} second".($s==1?'':'s'); } $hour = 60*60; // 3600 if( $_POST['seconds'] >= $hour ) { $h = intval($_POST['seconds']/$hour); $hh = $h < 10 ? "0$h" : $h; $m -= ($h*60);// = intval( ($_POST['seconds']-($h*$hour)) / $minute);//$minute); $mm = $m < 10 ? "0$m" : $m; $_POST['hours'] = "$hh:$mm:$ss<br>{$h} hour".($h==1?'':'s').", {$m} minute".($m==1?'':'s').", {$s} second".($s==1?'':'s'); } $day = 60*60*24; // 86400 if( $_POST['seconds'] >= $day ) { $d = intval($_POST['seconds']/$day); $dd = $d < 10 ? "0$d" : $d; $h -= ($d*24); $hh = $h < 10 ? "0$h" : $h; $_POST['days'] = "$dd:$hh:$mm:$ss<br>{$d} day".($d==1?'':'s').", {$h} hour".($h==1?'':'s').", {$m} minute".($m==1?'':'s').", {$s} second".($s==1?'':'s'); } $_POST['elapsed'] = array(); $_POST['elapsed'][] = "<div style='white-space:nowrap;'>{$_POST['seconds']} second".($_POST['seconds']==1?'':'s')."</div>"; if( $_POST['minutes'] ) { $_POST['elapsed'][] = "<div style='white-space:nowrap;'>{$_POST['minutes']}</div>"; } if( $_POST['hours'] ) { $_POST['elapsed'][] = "<div style='white-space:nowrap;'>{$_POST['hours']}</div>"; } if( $_POST['days'] ) { $_POST['elapsed'][] = "<div style='white-space:nowrap;'>{$_POST['days']}</div>"; } $_POST['timeElapsed'] = implode('<div style="height:.5em;"></div>',$_POST['elapsed']); } function ConformInputTime($tm) { if( ! preg_match('/\d/',$tm) ) { return false; } $ret = array(); $tm = trim($tm); $ta = preg_split('/\D+/',$tm); if( count($ta) == 2 ) { $ta[] = '00'; } if( count($ta) != 3 ) { return false; } $h=intval($ta[0]); $m=intval($ta[1]); $s=intval($ta[2]); $ret = array('h'=>$h,'m'=>$m,'s'=>$s); return $ret; } # function ConformInputTime() function ConformInputDate($dt) { if( ! preg_match('/\d/',$dt) ) { return false; } $ret = array(); $dt = trim($dt); if( preg_match('/^\d\d\d\d\-\d\d\-\d\d$/',$dt) ) { list($y,$m,$d) = explode('-',$dt); $y=intval($y); $m=intval($m); $d=intval($d); $ret = array('y'=>$y,'m'=>$m,'d'=>$d); } else { $ta = preg_split('/\D+/',$dt); if( count($ta) != 3 ) { return false; } $m=intval($ta[0]); $d=intval($ta[1]); $y=intval($ta[2]); $ret = array('y'=>$y,'m'=>$m,'d'=>$d); } if( $ret['m']>12 and $ret['d']<13 ) { $i = $ret['m']; $ret['m'] = $ret['d']; $ret['d'] = $i; } return $ret; } # function ConformInputDate() ?><!DOCTYPE html> <html lang="en"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Time Elapsed</title> <style type="text/css"> * { box-sizing:border-box; } html, body { font-size:100%; font-family:sans-serif; } td { vertical-align:top; } tr { height:1.75rem; } a { text-decoration:none; } input[type='text'], input[type='date'] { font-size:1rem; font-family:monospace; width:150px; border:1px solid #ccc; border-radius:.3em; padding:.1em 0 .2em .3em; } </style> </head> <body> <div style="display:table; margin:.5in auto 0 auto;"> <a href="https://www.willmaster.com/"><img src="https://www.willmaster.com/images/wmlogo_icon.gif" alt="logo" style="margin-right:12px;"></a><h3 style="display:inline-block; vertical-align:top; margin-top:0;">Time<br>Elapsed</h3> </div> <?php if($_POST['timeElapsed']): ?> <div style="display:table; border:1px dashed #ccc; padding:1rem; margin:20px auto;"> <div><b>Time Elapsed</b></div> <table border="0" cellpadding="0" cellspacing="0" style="margin-top:.7rem;"> <tr> <td style="padding-right:.25rem;">From:</td><td><?php echo($_POST['fPostTime']) ?></td> </tr><tr> <td style="padding-right:.25rem;">To:</td><td><?php echo($_POST['tPostTime']) ?></td> </tr><tr> <td style="padding-right:.25rem; vertical-align:top;">Elapsed:</td><td><?php echo($_POST['timeElapsed']) ?></td> </tr> </table> </div> <?php endif; ?> <form method="post" enctype="multipart/form-data" accept-charset="utf-8" action="<?php echo(htmlspecialchars($_SERVER['PHP_SELF'])); ?>"> <div style="display:table; border:3px solid #ccc; border-radius:1rem; padding:1rem; margin:20px auto;"> <div>Dates are optional (blank = today). Time is required (seconds optional).</div> <div style="display:table; border:2px solid #ccc; border-radius:.7rem; padding:.5rem; margin-top:.5rem;"> <div><b>From</b></div> <div style="display:inline-block; margin-right:.75rem;">Date: <input type="date" name="fDate" placeholder="mm/dd/yyyy"></div> <div style="display:inline-block;">Time: <input type="text" name="fTime" placeholder="hh:mm:ss"></div> </div> <div style="display:table; border:2px solid #ccc; border-radius:.7rem; padding:.5rem; margin-top:.5rem;"> <div><b>To</b></div> <div style="display:inline-block; margin-right:.75rem;">Date: <input type="date" name="tDate" placeholder="mm/dd/yyyy"></div> <div style="display:inline-block;">Time: <input type="text" name="tTime" placeholder="hh:mm:ss"></div> </div> <div style="margin-top:1em; text-align:center;"><input type="submit" name="submitter" value="Get Time Elapsed"></div> </div> </form> <p style="display:table; margin:1em auto .5in auto;"> Copyright 2022 <a href="https://www.willmaster.com/">Will Bontrager Software LLC</a> </p> </body> </html>
Copy the source code and save it as TimeElapsed.php
or other *.php
name that is suitable to you.
Upload TimeElapsed.php
to your server. Make a note of its URL.
To use TimeElapsed.php
, type it URL into your browser. You'll see a control panel similar to the above screenshot. (There are no calculation results right at the beginning.)
The dashboard expects a beginning time and an ending time.
Dates are optional. Because dates are used in the calculation section of the software, blank dates are assumed to be the current day.
The beginning and ending time is required, although the number of seconds is optional. When the seconds are missing, "0" is assumed. Hours, minutes, and optional seconds are separated with a ":" character.
Tap the "Get Time Elapsed" button and the calculation results will be displayed for you.
With this installed and ready at one of your domains, you can do quick calculations.
(This article first appeared with an issue of the Possibilities newsletter.)
Will Bontrager