Fade-in Image Swap
Image swaps tend to be abrupt. This article describes an implementation where the new image fades in.
A div
on the web page is dedicated to showing the image. New images in a swap are faded in within that div
.
A swap occurs when the site user taps a thumbnail image or other link that has a special onclick
attribute.
The fade-in is done with the CSS transition
property.
Because it is CSS-handled, it generally results in a smooth transition.
The transition
property can be used in many different ways and situations and for many different implementations. Thus, it could seem complicated. But it doesn't have to be.
In this article, you'll see how simple it can really be. To keep it simple, only the way the CSS transition
property is used here is addressed, not the myriad of other ways it can be applied.
A Live Demonstration
Below is a live demonstration for fade-in image swaps. The demonstration images are from the Terra page at the LightFocus.com website.
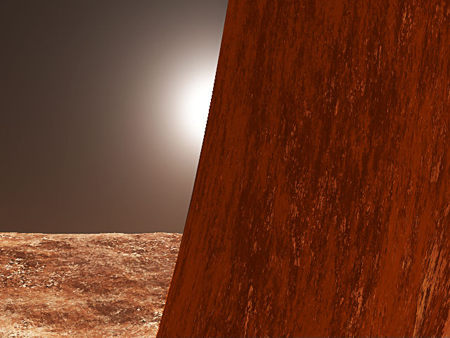
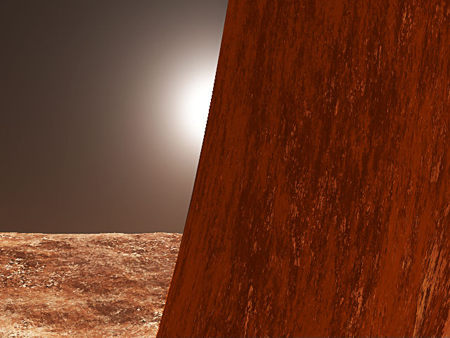
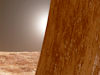
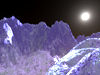
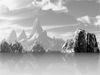
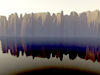
Tap on a thumbnail to swap in the larger image.
The thumbnails can be placed anywhere on the page. And the links don't have to be thumbnails, they can be text or a different image.
The fade-in transition for the demonstration is set to last 1 second. I'll show you how you can make your fade-in transitions faster or slower.
Implementing Fade-in Image Swap
Implementation requires 4 steps.
1. Where and What to Swap —
Determine where on your web page the feature will be.
Note the URLs of the images that will be swapped.
Also note the URLs of the thumbnails or other images that will be linked for doing image swaps. (Linked text may be used instead of images.)
2. Image Area —
The image area is contained in a div
. When an image is swapped, the image area fades in the new image and displays it until another image is swapped.
<div style="position:relative; height:338px;"><!-- Image swap area container. --> <div id="image-div-1" style="opacity:0; transition:opacity 1s linear; position:absolute; top:0; left:0; width:450px; height:338px;"> <img id="image-tag-1" src="" style="width:450px; height:338px;" alt="Pic"> </div> <div id="image-div-2" style="opacity:1; transition:opacity 1s linear; position:absolute; top:0; left:0; width:450px; height:338px;"> <img id="image-tag-2" src="https://lightfocus.com/terraimages/mars2-450.jpg" style="width:450px; height:338px;" alt="Pic"> </div> </div><!-- / Image swap area container. -->
You are likely to want to change a few things — unless you are putting this on a test page as-is to see how it works.
The CSS is inline and it is the minimum required. You may add other CSS. You may also put the CSS into an external style sheet.
The following might be changed:
-
The div on the very first line begins the container for the image swap area. You'll notice this CSS definition:
height:338px;
Change
338px;
to the height of the images that will occupy the container. -
Each of the next two divs contain an image tag. The
div
tag has anid
value and theimg
tag has anid
value. If you change any of theseid
values, then you will also need to change the JavaScript (see the next step). If you leave theid
values as they are, then the JavaScript won't need any customization. -
Each of those two
div
tags that contain animg
tag has this CSS declaration:transition:opacity 1s linear;
The
1s
part of that declaration tells the browser how much time to take to do the fading in when an image swap is made. The digit "1" in1s
specifies how many and the letter "s" in1s
specifies seconds. So1s
means 1 second.To change the fade-in speed, change
1s
to your preferred speed. Decimal numbers may be used. ".5s" (no quotes) would specify a ½ second fade-in. "2.5s" (no quotes) would specify 2½ seconds.Any fade-in speed change needs to be done in both places.
-
The
src
values of the image tags —-
The
img
tag in the first of the twodiv
tags has an emptysrc
value.src=""
That first image tag is open because no image swap has yet been made. When an image swap is made, the JavaScript will give the new image URL to the
src
attribute. -
The
img
tag in the second of those twodiv
tags has thissrc
value.https://lightfocus.com/terraimages/mars2-450.jpg
It is the image that will show within the image area when the page is first loaded. Change that
src
URL to the URL of your preferred image.
-
-
Each of the two
div
tags that contain animg
tag, and also each of theimg
tags themselves, has this CSS declaration:width:450px; height:338px;
That is the height and width of the images to be swapped in. If your images are a different size, change those declarations accordingly. You'll need to make the same change in 4 places.
-
https://lightfocus.com/terraimages/mars2-450.jpg
Replace the URL with the URL of the full-size image that will be swapped into the image area.
-
https://lightfocus.com/terraimages/thmars2.jpg
Replace the URL with the URL of the thumbnail or other image. If you are using linked text instead of an image, replace the entire
img
tag with the text to be linked. -
width:100px; height:75px;
Replace the dimensions with the dimensions of the thumbnail or other image. (If you are using linked text instead of an image, the dimensions would be removed when the entire
img
tag is replaced with the text to be linked.)
Place the image area on your web page where you want the images to be published.
3. JavaScript —
Here is the JavaScript for the fade-in image swap.
<script type="text/javascript">
/* Begin customization area. */
var ImageDiv1 = "image-div-1";
var ImageDiv2 = "image-div-2";
var ImageTag1 = "image-tag-1";
var ImageTag2 = "image-tag-2";
/* End of customization area. */
function ImageSwapThumbnailTapped(src)
{
if( document.getElementById(ImageDiv1).style.opacity == 1 )
{
document.getElementById(ImageTag2).src = src;
document.getElementById(ImageDiv1).style.opacity = 0;
document.getElementById(ImageDiv2).style.opacity = 1;
}
else
{
document.getElementById(ImageTag1).src = src;
document.getElementById(ImageDiv1).style.opacity = 1;
document.getElementById(ImageDiv2).style.opacity = 0;
}
}
</script>
If you did not change any of the id
values of the div
and img
tags for the image area (in the previous step), then the JavaScript won't need any customization.
Otherwise, if any of those have been changed, change the values in the customization section accordingly. The variable names indicate which id
value they expect.
The JavaScript will work from anywhere in the page. Unless there is a different place you prefer for it, the JavaScript may be put at the bottom of the page, immediately above the </body>
tag, so the rest of the page can load first.
4. Thumbnails —
Thumbnails for swapping an image can be anywhere on the page. Generally, they would be next to where the swapped-in image will be in a way that makes it intuitive what the thumbnail is for — but that is not required.
The thumbnail image can be any image or even plain text.
Here is the format for the thumbnail/image/text link.
<img onclick="ImageSwapThumbnailTapped('https://lightfocus.com/terraimages/mars2-450.jpg')" src="https://lightfocus.com/terraimages/thmars2.jpg" style="width:100px; height:75px;" alt="thumbnail">
In the format, replace:
You can add as many thumbnails as you wish, each swapping in a different image.
The thumbnails may be placed anywhere on the page. Placing them near the full-size image area would generally be prudent.
Notes
There isn't much testing to do, just tap on the thumbnail and verify the image gets swapped.
What you have is a smooth-transition image swap because it is handled with CSS. The JavaScript is used to tell the browser (and the CSS) which image to swap in next.
(This article first appeared with an issue of the Possibilities newsletter.)
Will Bontrager