Flip Content Front-Side to Back-Side
A div with content seems to flip and show the back side of the div. After a pause, it flips again to show the front side. And after another pause, it flips again.
The content can be images, text, or a combination. Actually, any content that can be contained in a div may be used.
To help visualize the potential, two examples are provided.
Here's the first one.
There are two images, both displayed in an oval div. The flip speed is fairly fast. The speed will depend on the speed of your browser or your computer/
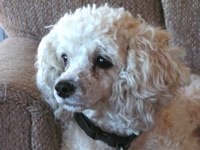
The example functionality pertains only to the oval images. The sign-holder simulation is extraneous, added to make the example more interesting.
When the content appears to flip, this is really what happens:
There are two divs. Only one div at a time is displayed.
The two divs are inside another div. This container div is used for the animation.
The container div's sides are pushed in, squeezing the content it contains, until the container div is less than 2 pixels in width.
At that point, the current content div is undisplayed and the other content div is displayed. Now, the container div's sides are expanded, revealing the content of the currently-displayed div.
The effect is a simulation of the content of the div flipping from its front side to it's back side. And then the reverse.
Here's the second example.
So it's easier to visually follow the transition, the flip speed of this second example is slower. The interval between flips is 5 seconds.
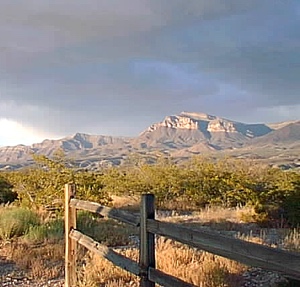
Here is the source code for the content flipper. You'll be able to adjust the flip speed and the interval between the flips. And there are other customizations available. Notes follow the source code.
<div id="flip-div-container" style="width:300px;"> <div id="animated-div" style="margin-left:0; margin-right:0; height:287px; overflow:hidden; border:1px solid #666; border-radius:50% 50%;"> <div id="front-side" style="display:block; height:287px;"> <img src="//www.willmaster.com/library/images/contentFlipper/old-west.jpg" style="height:287px; width:300px; border:none; outline:none;"> </div><!-- id="front-side" --> <div id="back-side" style="display:none; height:287px;"> <img src="//www.willmaster.com/library/images/contentFlipper/inthegarden.jpg" style="height:287px; width:300px; border:none; outline:none;"> </div><!-- id="back-side" --> </div><!-- id="animated-div" --> </div><!-- id="flip-div-container" --> <script> /* Flip Content Front-Side to Back-Side Version 1.0 July 11, 2016 Will Bontrager Software LLC https://www.willmaster.com/ Copyright 2016 Will Bontrager Software LLC This software is provided "AS IS," without any warranty of any kind, without even any implied warranty such as merchantability or fitness for a particular purpose. Will Bontrager Software LLC grants you a royalty free license to use or modify this software provided this notice appears on all copies. */ /* Begin customization (see Willmaster.com library article). */ var TheContainerID = "flip-div-container"; var TheAnimatedDivID = "animated-div"; var FrontSideContentDivID = "front-side"; var BackSideContentDivID = "back-side"; var FlipInterval = 5000; var FlipSpeed = 10; /* End customization. */ var AnimatedDiv = document.getElementById(TheAnimatedDivID); var MaxMargin = parseInt( parseInt(document.getElementById(TheContainerID).style.width) / 2 ) - 1; var One = document.getElementById(FrontSideContentDivID); var Two = document.getElementById(BackSideContentDivID); var Margin = 0; function FlipTheDiv() { Margin++; AnimatedDiv.style.marginLeft = Margin + "px"; AnimatedDiv.style.marginRight = Margin + "px"; if( Margin < MaxMargin ) { setTimeout(FlipTheDiv,FlipSpeed); } else { if( One.style.display == "none" ) { One.style.display = "block" Two.style.display = "none"; } else { One.style.display = "none" Two.style.display = "block"; } Opening = true; FinishTheFlip(); } } // function FlipTheDiv() function FinishTheFlip() { Margin--; AnimatedDiv.style.marginLeft = Margin + "px"; AnimatedDiv.style.marginRight = Margin + "px"; if( Margin > 0 ) { setTimeout(FinishTheFlip,FlipSpeed); } else { setTimeout(FlipTheDiv,FlipInterval); } } // function FinishTheFlip() setTimeout(FlipTheDiv,FlipInterval); </script>
Source code notes —
The source code has colored sections:
-
There are four divs, each with an id value. The id values in the div tag and the same values in the JavaScript are colored green.
-
Some of the inline CSS rules assigned to the divs are important and others are optional. The important rules are colored red. The optional rules have no special coloring and may be removed or changed. Other CSS rules may be added.
-
The JavaScript values that specify the timing are colored blue.
The customization for the divs is within the div tags. The customization section for the JavaScript is about 20 lines into the JavaScript, immediately below the information header section.
You can do a lot with the content flipper. Here are the customization details.
The Divs
There are four divs.
-
The Container Div contains the other three divs. This div is used to position the flipping content and to restrain its width.
-
The Animated Div contains the two content divs. Its animation comes from JavaScript increasing and decreasing the div's left and right margins, which pushes the div's sides in or lets them out. The Container Div provides the edges or walls against which the margins rest as they increase or decrease.
-
The Content Front Side Div contains the content for the front side. It's either displayed or not displayed, the opposite of the Content Back Side Div.
-
The Content Back Side Div contains the content for the back side. It's either displayed or not displayed, the opposite of the Content Front Side Div.
The Container Div — Its id value flip-div-container is specified at the TheContainerID
variable in the customization section of the JavaScript.
It's only CSS rule of importance is width:300px; that you'll customize to what your implementation requires. The width is the maximum width of the flipping content. The rule may be moved to a style sheet.
The Animated Div — Its id value animated-div is specified at the TheAnimatedDivID
variable in the customization section of the JavaScript.
It has 4 important CSS rules, margin-left:0; margin-right:0; height:287px; overflow:hidden;. The rules may be moved to a style sheet.
-
margin-left:0; is required as is. The JavaScript will change this value as needed to contract and expand the Animated Div.
-
margin-right:0; is required as is. The JavaScript will change this value as needed to contract and expand the Animated Div.
-
height:287px; needs to be customized. The height is the exact height of the content divs.
-
overflow:hidden; is required as is. It prevents the content from spilling out the sides as the Animated Div contracts and expands.
As you see, only one of the Animated Div important inline CSS rules needs to be customized, the height:287px; rule.
The Content Front Side Div — Its id value front-side is specified at the FrontSideContentDivID
variable in the customization section of the JavaScript.
It has 2 important CSS rules, display:block; height:287px;. The height:287px; rule may be moved to a style sheet but the display:block; rule needs to be inline.
-
display:block; is required as is. The rule ensures this front side is displayed when the web page first loads.
-
height:287px; needs to be customized. The height value needs to be the same as the height value assigned to the Animated Div.
The example content is an image that covers the entire front side div. If your implementation has an image to cover the entire div, then the height and the width of the image must match exactly the height of the Animated Div and the width of the Container Div.
The Content Back Side Div — Its id value front-side is specified at the BackSideContentDivID
variable in the customization section of the JavaScript.
It has 2 important CSS rules, display:none; height:287px;. The height:287px; rule may be moved to a style sheet but the display:none; rule needs to be inline.
-
display:none; is required as is. The rule ensures this back side will not be displayed when the web page first loads.
-
height:287px; needs to be customized. The height value needs to be the same as the height value assigned to the Animated Div.
Like the front side div, the example content is an image that covers the entire back side div. If your implementation has an image to cover the entire div, then the height and the width of the image must match exactly the height of the Animated Div and the width of the Container Div.
When the div customization is done, you're ready to customize the JavaScript.
The JavaScript
There are six places to customize, all in the customization area beginning about 20 lines from the top of the JavaScript, below the header information area.
The first four are where the div ID values are assigned. The last two are where the interval and speed of the flips are assigned.
The first four JavaScript customizations.
Assign the ID values of the divs to the four JavaScript variables. The variable names tell you which div ID to assign. To make things clear, the green colored values in the JavaScript code match the green colored ID values of the divs.
The last two JavaScript customizations.
Assign values to the last two variables according to this:
-
The variable
FlipInterval
is where you tell the JavaScript how many milliseconds to pause between each flip. The code has 5000 milliseconds assigned, which is 5 seconds. Change the value as your implementation requires. -
The variable
FlipSpeed
is where you tell the JavaScript how many milliseconds to pause between each flip contraction or expansion increment. Every increment either expands or contracts the margin on each side of the Animated Div by 1 pixel. The code has 10 milliseconds assigned. For comparison, the first example in this article has aFlipSpeed
of 5 milliseconds and the second example has aFlipSpeed
of 100 milliseconds.
That's it for the customization. All that's left is to put it on a web page and test it.
The Installation
Paste the code you customized into your web page where you want the flipping content to be published.
The JavaScript may be immediately below the divs or it may be lower on the page. It may also be imported from an external file.
As stated earlier, the divs with content to be flipped may contain images or text by themselves or in any combination.
(This article first appeared in Possibilities ezine.)
Will Bontrager