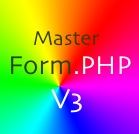
User Manual
General | Control Panel | Form Features | ||
---|---|---|---|---|
Confirmation/Thank-you
Page Customization
Index
- Required PHP Code
- Optional Customization
- Publish form error messages
- Publish certain content only if there were no error messages
- Customize page with information from the form
- Customize conditionally
- If the form field is empty
- If the form field contains any information
- If the form field contains specific information
- If the form field's value equals 1 or is greater than 1
- Customize with calculations on form field values
Required PHP Code
The confirmation/thank-you page needs to be a PHP web page. In the page source code, insert this PHP code:
<?php $InstallationDirectory = "/installed"; include($_SERVER["DOCUMENT_ROOT"]."$InstallationDirectory/MasterFormInclude.php"); ?>
Put the PHP code into the head area of the web page source code, if possible. Otherwise, as high in the body area as is feasible.
The higher in the web page source code that the line is inserted, the better. Customization can occur only below the point where the line is inserted.
Optional Customization
The following customization features are optional:
-
Publish form error messages with or without related content.
-
Publish certain content only if there were no error messages.
-
Customize page with information from the form.
-
Conditionally customize — if certain information was provided on the form, publish certain content; otherwise, publish other content.
-
Customize page with the results of arithmetic calculations on form field values.
Publish form error messages.
To insert form use error messages, put this in the
<?php echo($ErrorMessage); ?>
When there are any error messages, they will print. When there are no error messages, nothing extra will print.
Formatting the error message:
The error messages are in a div with id="masterform_errorbox" and the messages themselves are in a UL list.
The following CSS definitions will put 5 pixels of margin below each message, print the messages text 14 pixels in size, bold, and red, and remove the underline of any links in the messages:
<style type="text/css"> #masterform_errorbox ul, li { margin:0 0 5px 0; } #masterform_errorbox li { font-size:14px; font-weight:bold; color:red; } #masterform_errorbox a { text-decoration:none; } </style>
A border around the error message:
To put a border around the message, create a div and put the <?php echo($ErrorMessage); ?> into the div. In that case, put the div in a PHP if() tag so the div will print only if an error message is available.
The following will print a div with a border, a statement, the error message(s), and another statement only if an error message is available:
<?php if( $ErrorMessage ): ?> <!-- Error message is in div with id="masterform_errorbox" --> <style type="text/css"> #masterform_errorbox ul, li { margin:0 0 5px 0; } #masterform_errorbox li { font-size:14px; font-weight:bold; color:red; } #masterform_errorbox a { text-decoration:none; } </style> <div style="border:1px dashed black; padding:0 25px 0 25px; margin:25px;"> <p> Message for you: </p> <?php echo($ErrorMessage); ?> <p> <a href="javascript:history.go(-1)">Click here</a> or use your browser's "Back" button to return to the form. </p> </div> <?php endif; ?>
<?php if( $ErrorMessage ): ?> starts the block and it ends with <?php endif; ?> (Note that if(...) is followed with a colon and endif is followed with a semi-colon.)
Everything between those two tags will print only if $ErrorMessage contains a value.
Publish certain content only if there were no error messages.
If your confirmation/thank-you page contains a "Thank you! We'll send that to you right away." message, you don't want to print it if the form user forgot to type in their email address.
Here is how to omit certain content from the page if there are any error messages.
<?php if( ! $ErrorMessage ): ?> <h1>Thank you!</h1> <p>We'll send that to you right away.</p> <?php endif; ?>
<?php if( ! $ErrorMessage ): ?> starts the block (the "!" means "not") and it ends with <?php endif; ?>
Customize page with information from the form.
Here is the code to print the value of any form field into the
Edit the code per the following note. Then, paste it into the web page source code where the information is to be published.
Note: In the above code, replace FIELDNAME with the form field name of the value to publish. Field names are case sensitive.
Example:
<p> An email has been sent to your <?php echo(@$_POST["email"]); ?> address. </p>
Any form field names may be used to customize the
Customize conditionally.
Specific content can be published or not published depending on whether or not certain form fields contain information, or if they contain certain information.
A form field is referenced with $_POST["FIELDNAME"] where FIELDNAME is the form field name. Form field names are case-sensitive.
Four examples follow.
1. If the form field is empty (the field contains no information or the field does not exist):
The example code checks if the form field name="telephone" is blank (or doesn't exist). If the field is empty, a "We can't call you without your telephone number!" message is printed.
<?php if( empty($_POST["telephone"]) ) { echo "<p>We can't call you without your telephone number!</p>"; } ?>
The message is printed with the echo command. The message itself is between quotation marks. (If the message contains quotation marks within itself, escape them with a preceding backslash character. Like: \"
2. If the form field contains any information:
The example code checks if the form field name="telephone" contains information. If it does, a message with the telephone field value is printed.
<?php if( ! empty($_POST["telephone"]) ) { echo "<p>Telephone: " . $_POST["telephone"] . "</p>"; } ?>
The message between quotation marks is parted where the form field value is inserted. This is the format:
"message part " . VALUE . " another message part"
Each message part is between quotation marks. A period between the form field value to be inserted between the message parts ties them together. The value in the prior example is $_POST["telephone"] (the form field name also between quotation marks).
3. If the form field contains specific information:
This code checks that form field name="name" exists and contains the value Tom (exactly). If it does, a message is printed.
<?php if( @$_POST["name"] == "Tom" ) { echo "<p>Sorry, no \"Tom's\" allowed!</p>"; } ?>
The "@" character at the start of @$_POST["name"] is a special symbol that results in null or "" if the field being checked does not exist.
If the form field's value is "Tom", the message between the quotation marks is printed. Notice that the quotation marks within the message are escaped with a backslash character.
4. If the form field's value equals 1 or is greater
If form field name="quantity" exists and equals 1 or a number greater than one, print the message.
<?php if( @$_POST["quantity"] >= 1 ) { echo "<p>Quantity: " . $_POST["quantity"] . "</p>"; } ?>
The above is PHP code used to check form fields' existence and value. Then the check used to determine whether or not a certain message is to be printed.
PHP code can be used to check any form fields using the above techniques and other techniques PHP makes available.
Customize with calculations on form field values.
Arithmetic calculations can be done on form field values with form field names in PHP code.
Here are two example placeholders, one with an addition operating and the other a division:
<?php echo($_POST['Item1'] + $_POST['Item2']); ?> <?php echo($_POST['Item1'] / $_POST['Item2']); ?>
Assuming form field name="Item1" has a value of 33 and field name="Item2" has a value of 7, then those two placeholders will be replaced with:
40 4.71428571428571
Division accuracy is limited by what your installation of PHP and your Internet server software can utilize when doing calculations.
The calculated result can be rounded to a certain number of decimal places.
To accomplish that, use the Decimalize() function.
Call the Decimalize() function with the calculation and the number of decimal places to round to. (Rounding is to the nearest number.
For two decimal places 1.001 would be rounded to 1.00 and 1.005 would be rounded
Here are the same examples except instructing the results be rounded to 2 decimal places:
<?php echo( Decimalize($_POST['Item1'] + $_POST['Item2'], 2) ); ?> <?php echo( Decimalize($_POST['Item1'] / $_POST['Item2'], 2) ); ?>
Assuming the field names have the same values as before, those placeholders would be replaced with:
40 4.71
The software can be instructed to append 0's to force the result to have the same number of decimal places as the rounding specified.
To accomplish that, send a third value, true (no quotes) to the Decimalize() function.
Here are the same examples except a true value to force the result to have the number of decimal places as specified with the rounding digit:
<?php echo( Decimalize($_POST['Item1'] + $_POST['Item2'], 2, true) ); ?> <?php echo( Decimalize($_POST['Item1'] / $_POST['Item2'], 2, true) ); ?>
Assuming the field names have the same values as before, those placeholders would be replaced with:
40.00 4.71
To round to the nearest whole number, use the Decimalize() function and specify 0 (zero) as the number of decimal places to round to.
<?php echo( Decimalize($_POST['Item1'] + $_POST['Item2'], 0) ); ?> <?php echo( Decimalize($_POST['Item1'] / $_POST['Item2'], 0) ); ?>
Assuming the field names have the same values as before, those placeholders would be replaced with:
40 5
Rounding accuracy is limited by the number of decimal places your installation of PHP and your Internet server software can utilize when doing calculations.
The following mathematical symbols may be used in the placeholder:
Symbol | Operation |
---|---|
+ | addition |
- | subtraction |
* | multiplication |
/ | division |
% | remainder |
Calculations may include parenthesis to force calculation of certain parts of the equation before other parts. The part between the parenthesis will be calculated first.
Here are two examples:
<?php echo( ($_POST['Item1'] / $_POST['Item2']) + $_POST['Item2'] ); ?> <?php echo( $_POST['Item1'] / ($_POST['Item2'] + $_POST['Item2']) ); ?>
Assuming form field name="Item1" has a value of 33 and field name="Item2" has a value of 7, then those two placeholders will be replaced with:
11.7142857142857 2.35714285714286
In the first placeholder, 33 is divided by 7, then 7 is added. In the second placeholder 7 is added to 7, resulting in 14, then 33 is divided by 14 .
Numbers can be included in the operation in addition to or instead of form field names. Here are several examples:
<?php echo( ( ($_POST['Item1']/$_POST['Item2']) + $_POST['Item2'] ) - 9); ?> <?php echo( 44 / (2 + 4) ); ?> <?php echo( $_POST['Item1'] * $_POST['Item2'] ); ?> <?php echo( $_POST['Item1'] / $_POST['Item2'] ); ?> <?php echo( $_POST['Item1'] % $_POST['Item2'] ); ?>
Assuming the values of the form fields are as before, the above placeholders would be replaced with:
2.71428571428572 7.33333333333333 231 4.71428571428571 5
The CountNonEmptyFields() function to determine the number of form fields that have check marks or otherwise contain information.
To obtain a count, use the CountNonEmptyFields() and specify a comma-separated list of form field names. Put the list between quotation marks. Example:
CountNonEmptyFields("Item1, Item2, Item8")
As an example of use, let's suppose you sold certain types of documents at $1.25 each. Your order form lists the documents next to checkboxes customers can check to buy. The form field names of the checkboxes are name="documents[]".
This example shows one way to present the total amount of the order, including an order tax of 6.5%.
<pre style="border:1px black solid. padding:10px;> <?php echo( "Number of documents: " . CountNonEmptyFields("documents") . " Cost of documents: " . Decimalize( CountNonEmptyFields("documents") * 1.25, 2) . " Tax on documents: " . Decimalize( (CountNonEmptyFields("documents")*1.25) * 0.065, 2) . " Total cost: " . Decimalize( ( (CountNonEmptyFields("documents")*1.25) * 0.065 ) + (CountNonEmptyFields("documents")*1.25), 2) ); ?> </pre>
For similar features to customize the email with placeholders, see the Email Placeholders User Manual section.
Master Form PHP V3, version 3.0
Copyright 2010, 2011 Bontrager Connection, LLC
Copyright 2011-2013, 2015, 2017, 2020 Will Bontrager Software LLC