Simple Image Lightbox
A few days ago, a project required a simple way to launch a fill-sized image when a thumbnail image was tapped.
The full-sized image was to be centered on the page on top of a partially transparent full-screen background. The image dimensions needed to be responsive to the screen size, but not larger than the natural dimensions of the image file. And the display needed to go poof with a tap anywhere on the page.
The full-sized image centered on a partially transparent full-screen background is a "lightbox" for some people. It's a good term. I'll use it in this article.
A version of the code developed for that project is in this article.
This is how simple it is to implement:
-
Paste some JavaScript on the page where the lightbox will be launched.
The JavaScript is copy and paste. Optionally, you may specify a different background color or opacity for the lightbox. (The default is the same as the lightbox examples on this page.)
-
The image that will launch the lightbox has an onclick attribute that calls the JavaScript function. The function is called with the URL of the image to display in the lightbox.
That's it. It really is that easy. And simple.
It's why I call it Simple Image Lightbox.
The JavaScript is further below. And examples — both live examples and example code.
Live Simple Image Lightbox Examples
These two thumbnail images will launch a larger version of the image displayed in a lightbox.
Generally, the lightbox is launched when an image thumbnail is tapped. However, the lightbox also can be launched with a link. And with a button or any other tappable HTML tag that can use an onclick
attribute.
Here are two link and two button examples that will each launch the lightbox.
Puppy lightbox Butterfly lightbox
The JavaScript
I'm putting the JavaScript here so it can be referenced when we talk about the code for the above examples.
<script type="text/javascript">
var WillMaster_ImageBoxNode;
function WillMaster_MakeImageBox(url)
{ /* Will Bontrager Software LLC */
/* Simple Image Lightbox */
/* Version 1.0 */
/* January 18, 2020 */
/* Customization section. */
// Variable BackgroundColor needs to be assigned
// the complete color for the background behind
// the image in the lightbox. The value may be
// rgba() or hsla() with valid parameters.
// Examples: rgba(0,0,0,.5) and hsla(0,0%,0%,.5)
var BackgroundColor = "rgba(0,0,0,.5)";
/* End of customization section. */
var DivIDvalue = "WillMaster_ImageBoxID";
if( url == DivIDvalue )
{
document.body.removeChild(WillMaster_ImageBoxNode);
return;
}
WillMaster_ImageBoxNode = document.createElement("div");
WillMaster_ImageBoxNode.id = DivIDvalue;
WillMaster_ImageBoxNode.style.width = "100vw";
WillMaster_ImageBoxNode.style.height = "100vh";
WillMaster_ImageBoxNode.style.backgroundColor = BackgroundColor;
WillMaster_ImageBoxNode.style.position = "fixed";
WillMaster_ImageBoxNode.style.left = "0";
WillMaster_ImageBoxNode.style.top = "0";
WillMaster_ImageBoxNode.setAttribute("onclick",arguments.callee.name+"('"+DivIDvalue+"')");
WillMaster_ImageBoxNode.innerHTML='<img src="'+url+'" style=" border:none; outline:none; max-width:100%; max-height:100%; position:absolute; top:50%; left:50%; transform:translate(-50%,-50%);" alt="lightbox image">';
document.body.appendChild(WillMaster_ImageBoxNode);
}
</script>
Copy the JavaScript and paste it on the page that will have the lightbox. No customization is required.
If you decide to change the background color or opacity, replace rgba(0,0,0,.5)
with the rgba()
values you desire. Alternatively, the hsla()
function may be specified instead of the rgba()
function. (For reference, the MDN Web Docs <color> page talks about colors, including the rgba()
and hsla()
functions.)
Implementing Simple Image Lightbox
It is assumed you have the above JavaScript pasted into a test web page. That's the main part since it is the code that launches the lightbox.
To launch the lightbox, you need to have the URL of the image to place into the lightbox
With the image URL, you can use the href or onclick attributes to launch the lightbox with that particular image in it.
Because it is most likely how you will use this, let's first show how to launch the lightbox by tapping an image thumbnail.
This is an image tag to publish a thumbnail image:
<img src="https://www.willmaster.com/possibilities/images/poss1069dog-250.jpg" style="max-width:100%; height:auto; border:none; outline:none;" alt="puppy thumbnail">
The above is one of the example thumbnail images. Change the image URL to your own thumbnail.
To launch the lightbox when the thumbnail is tapped, insert an onclick
attribute:
<img
onclick="WillMaster_MakeImageBox('https://www.willmaster.com/possibilities/images/poss1069dog-640.jpg')"
src="https://www.willmaster.com/possibilities/images/poss1069dog-250.jpg"
style="max-width:100%; height:auto; border:none; outline:none;"
alt="puppy thumbnail">
The above onclick
uses one of the example lightbox images. Change the image URL to your own image.
That is all the coding you need to do. Here is the result.
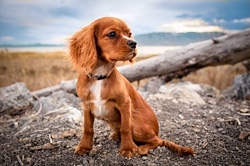
For comparison, here is the code for the butterfly example.
<img
onclick="WillMaster_MakeImageBox('https://www.willmaster.com/possibilities/images/poss1069butterfly-640.jpg')"
src="https://www.willmaster.com/possibilities/images/poss1069butterfly-250.jpg"
style="max-width:100%; height:auto; border:none; outline:none;"
alt="butterfly thumbnail">
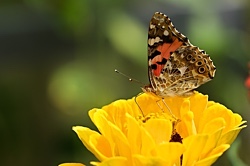
Using links to launch Simple Image Lightbox
To use a link for launching the lightbox, specify javascript:
followed by the function name and image URL. Here is example code for the puppy image.
<a
href="javascript:WillMaster_MakeImageBox('https://www.willmaster.com/possibilities/images/poss1069dog-640.jpg')"
style="white-space:nowrap;">
Puppy lightbox
</a>
Change the lightbox image URL in the href
attribute and update the link text. Then you're good to go.
Working example of above code: Puppy lightbox
Using a button or other HTML tags to launch Simple Image Lightbox
For buttons and other tappable HTML tags that can use the onclick
attribute, use the same onclick
value as a thumbnail image would take. Here is example code for the puppy image.
<input
type="button"
onclick="WillMaster_MakeImageBox('https://www.willmaster.com/possibilities/images/poss1069dog-640.jpg')"
value="Puppy Lightbox">
Change the lightbox image URL and the link text and you're good to go.
Working example of above code:
Use the onclick
attribute for any other HTML tags. Examples are divs or table data cells containing any content.
To review, put the lightbox-launching JavaScript into the web page. Then call the JavaScript function when a thumbnail image is tapped. The lightbox also may be launched with a link. In addition, HTML tags that can use the onclick
attribute, like buttons, can be used to launch the lightbox.
(This article first appeared with an issue of the Possibilities newsletter.)
Will Bontrager